A student record system is a platform for managing student information. Regardless of being a paper-based or automated system, student record system yields a lot of benefits to the school. The system provides crucial information, which can be very helpful in making decisions concerning the management of the institution. The student record can be integrated with the school information management system and improve efficiency for the general management of the school activities.
The main functions included in a student record system are courses registration, generating reports, results capturing and computation and data analysis. Using the techniques in this course, ‘fundamentals of programming’, I am going to design and implement a simple module where students’ can enter the grades and compute the average grades. The system is implemented using visual studio windows application in C# programming language. The system implementation employs the concepts of object oriented programming language, including classes, objects, and methods. The system also uses the application of control structures among other programming concepts.
Design and Coding
The system has two pages, a welcome page and grade capturing page where the student can enter the grades and compute the average grade. The Grade Class has one method called getGrade, which fecthes the grade, assigns it some points, and stores it in integer variable pts. To assign the grade point, I have used the if statement. The if statement is a decision making statement used to control the flow of execution of a code segment. It is a two-way statement used in conjunction with a test expression, using the syntax: If (test expression). The test expression is first tested during execution then passes the control to a particular statement depending on whether the expression control is true or false. The main code of the system is as shown below.
class Grade //create a glass call grade
{
public void getGrade (string grde, int pts)
{
try
{
if (grde==”A”) {pts = 12;}
if (grde == “A-“) {pts = 11;}
if (grde == “B+”) {pts = 10;}
if (grde == “B”) {pts = 9;}
if (grde == “B-“) {pts = 8;}
if (grde == “C+”) {pts = 7;}
if (grde == “C”) {pts = 6;}
if (grde == “C-“) {pts = 5;}
if (grde == “D”) {pts = 4;}
if (grde == “D”) {pts = 3;}
if (grde == “D-“) {pts = 2;}
if (grde == “D-“) {pts = 1;}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
}
}
The following code shows the calling of the class method. The method getGrade is called during the button click event or when a dropdown list is selected.
Grade obj = new Grade();
obj.getGrade(cbGrade.Text,pts);
When a student enters a grade, the student clicks the add button to add the grade into an array of grades and assigns points to the grade. The following shows the add button vent.
private void btnAdd_Click (object sender, EventArgs e)
{
try
{
getPoints();
//call inbuilt datatable class and an object table
DataTable table = new DataTable();
//add the grades and points the table object
table.Rows.Add(cbGrade.Text, pts);
gdGrades.DataSource = table;
//compute the total points and assign them to the variable totalPoints
totalPoints = totalPoints + pts;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
}
}
After adding all the grades entered into the data table, the user can call the compute average method which computes the average grade and displays it in a label. The code is as shown below.
public void computeAverage()
{
try
{
avgPts = totalPoints / gdGrades.Rows.Count
if (avgPts == 12)
{txtAverageGrade.Text = “A”;}
if (avgPts == 11)
{txtAverageGrade.Text = “A-“;}
if (avgPts == 10)
{txtAverageGrade.Text = “B+”;}
if (avgPts == 9)
{txtAverageGrade.Text = “B”;}
if (avgPts == 8)
{txtAverageGrade.Text = “B-“;}
if (avgPts == 7)
{txtAverageGrade.Text = “C+”;}
if (avgPts == 6)
{txtAverageGrade.Text = “C”;}
if (avgPts == 5)
{txtAverageGrade.Text = “C-“;}
if (avgPts == 4)
{txtAverageGrade.Text = “D+”;}
if (avgPts == 3)
{txtAverageGrade.Text = “D”;}
if (avgPts == 2)
{txtAverageGrade.Text = “D-“;}
if (avgPts == 1)
{txtAverageGrade.Text = “E”;}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
}
Error Handling
To handle errors with the program, I have employed the try catch exception concept. The block of code to be tested is placed inside the try block. The statement to be executed is placed in the try block and if an error occurs in the try block, it is placed in the catch statement (Lauwens, 2017). The try and catch code is shown below.
try
{
Grade obj = new Grade ();
obj.getGrade(cbGrade.Text,pts);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
Interface Screenshots
The first screen, Fig 1, is the welcome screen, on clicking capture grades button; it takes you to the capture grade page, Fig. 2. The second screen, Fig. 2, is the grade-capturing screen where the students can enter the grades and compute the average. You can capture as many grades and compute the average grade. After selecting a grade, the student enters a score or marks in the Points textbox, and clicks on Add to go to the next grade item. Clicking on the Clear button clears everything to leave the form empty and allow multiple use of the system. The use can click on the exit button to return to the welcome screen.
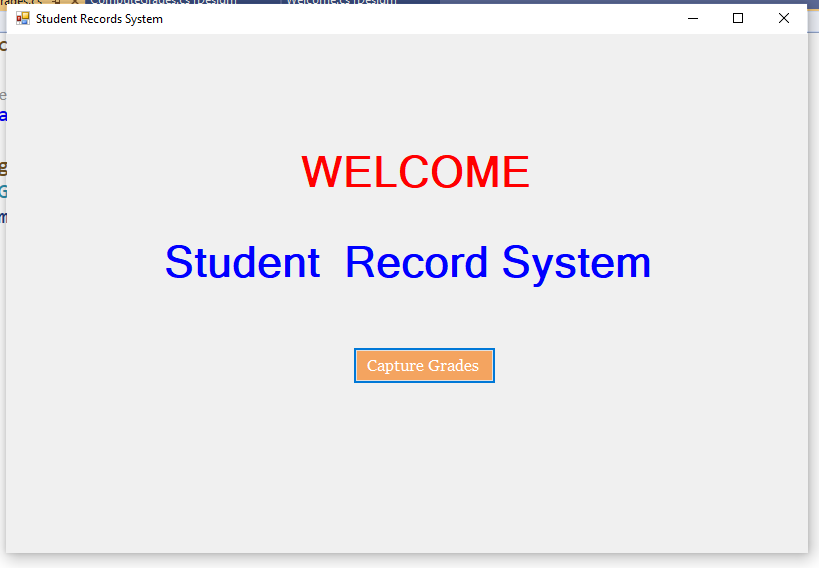
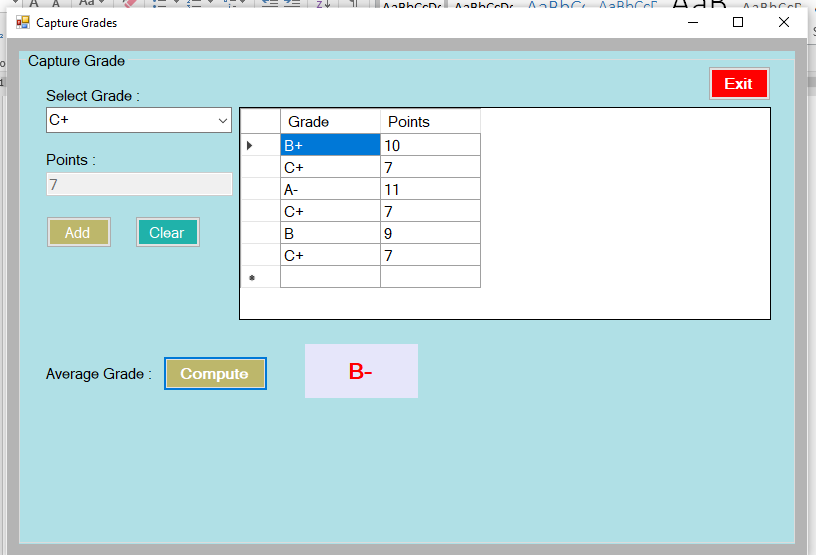
Reference
Lauwens, B. (2017) ‘ResumableFunctions: C# sharp style generators for Julia,’ Journal of Open Source Software, 2(18), p. 400. Web.